Speeding up CORS preflight requests with Cloudflare Workers
As a security measure browsers perform CORS preflight requests, but sometimes those preflight requests add significant latency - for example if your CORS-enabled API is hosted in single location and you have users all across the world. Some users located far away from your API server might need to wait extra 200-300ms to just get CORS response.
This can be improved by routing all OPTIONS
requests to Cloudflare Worker that should respond
quickly no matter where your users are.
In my case it didn’t make sense to route all API traffic through Cloudflare worker (which
can be done using its fetch
method). With suggested solution only OPTIONS
requests
are handled by the worker, other traffic is handled directly by the API.
Following diagram shows what we are trying to achieve:
With this setup we get benefit of faster CORS requests, but at the same time we are not adding extra latency by proxying every request.
Cloudflare doesn’t have direct way to connect worker just for specfiic HTTP method, so we need to workaround it using page rules.
Step 1. Create worker
First you need to create Cloudflare Worker. It doesn’t need to have custom domain as we won’t be using it directly.
This is simplest version that just returns Access-Control-Allow-Origin
header.
const corsHeaders = {
"Access-Control-Allow-Origin": "*",
"Access-Control-Allow-Methods": "GET,HEAD,PUT,PATCH,POST,DELETE",
"Access-Control-Allow-Headers": "*",
};
export default {
async fetch(request, env, ctx) {
if (request.method === "OPTIONS") {
return new Response(null, {
status: 204,
headers: corsHeaders,
});
}
return new Response("Proxy is working. Will respond to OPTIONS");
},
};
To create worker in Cloudflare account dashboard:
- Go to Compute (Workers).
- Click Create.
- Select Hello World template.
- Pick some worker name and click Deploy.
- Click Edit code.
- Use worker code from above and click Deploy.
Step 2. Create rule to redirect OPTIONS
requests to /cors-proxy
We will redirect all OPTIONS
requests to some path on your domain. Exact path isn’t
really important as we will just use it to connect worker in later steps.
In Cloudflare dashboard for you domain:
- Go to Rules.
- Click Create rule.
- Select URL Rewrite Rule.
- Use following rule details:
- Rule name: Rewrite OPTIONS request to /cors-proxy
- If incoming requests match…: Custom filter expression
- Use two fields with AND operator:
- Hostname - equals - api.example.com
- Request Method - equals - OPTIONS
- Then…:
- Path - rewrite to - static -
/cors-proxy
- Query - preserve
- Path - rewrite to - static -
- Click Save.
With this change all OPTIONS
requests will be forwarded to api.example.com/cors-proxy
.
This isn’t really useful on itself, but with this rule we can now connect our worker
to this path.
Step 3. Create Worker Route to connect your worker
Again, in Cloudflare dashboard for your domain:
- Go to Workers Routes.
- Click Add route.
- Fill it in as:
- Route:
api.example.com/cors-proxy
- Worker: Select worker you created in step 1.
- Route:
- Click Save.
Step 4. Enjoy faster CORS requests
You can now enjoy the results. Because preflight requests don’t ever hit your actual API and are served by worker you will get good response times for those requests regardless where you access it from as they run on Cloudflare’s edge network.
Before:
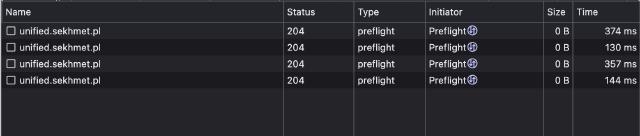
After:
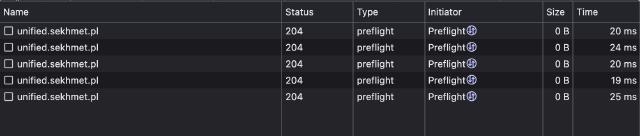